Producer-Consumer in Go: Beyond the Basics
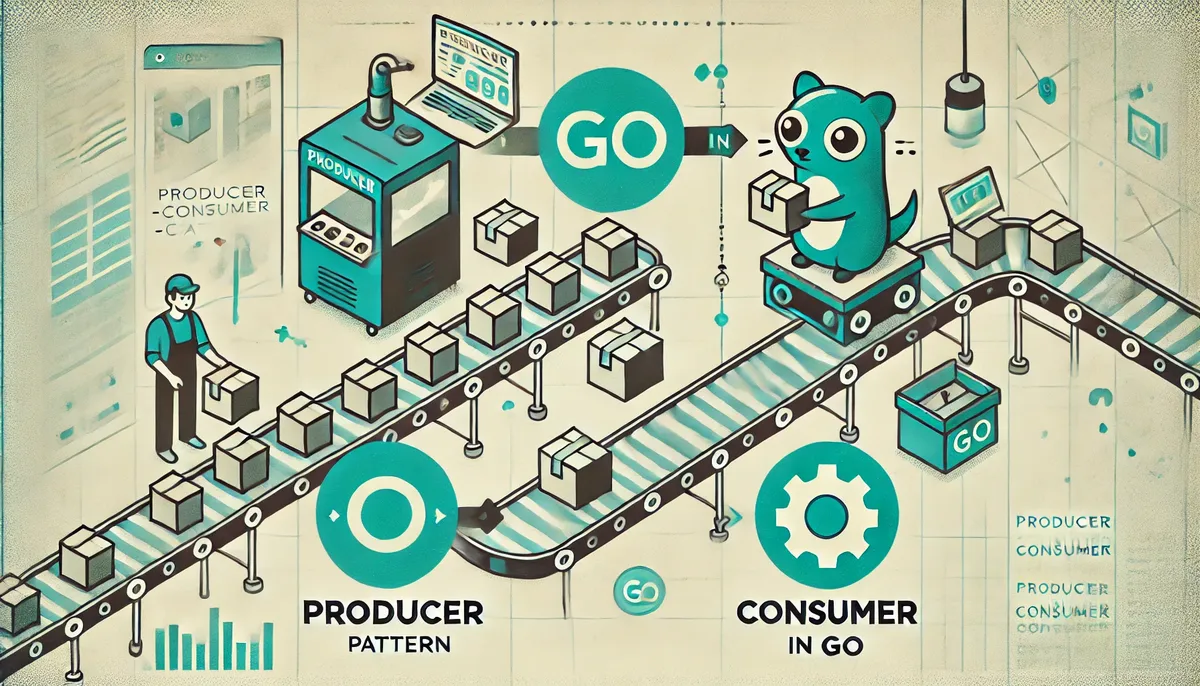
Producer-Consumer in Go: Beyond the Basics
Difficulty Level: IntermediateIn our previous article, we explored the fundamentals of the Producer-Consumer pattern in Go. Today, we’ll take it a step further by examining more practical scenarios and introducing buffered channels - a powerful feature that can significantly improve your concurrent applications.
Buffered Channels: A Game Changer
While regular channels provide immediate synchronization between producers and consumers, sometimes we need more flexibility. Buffered channels act like a small warehouse, temporarily storing items when producers are faster than consumers or when we want to batch process items.
Let’s see how they work:
// Unbuffered channel - synchronous
ch := make(chan int)
// Buffered channel - can hold up to 5 items
bufferedCh := make(chan int, 5)
The key difference? With a buffered channel, producers can send up to 5 items without waiting for a consumer to receive them. This can significantly improve performance in certain scenarios.
Real-World Example: Log Processing System
Imagine building a log processing system for a busy web application. Logs come in rapidly during peak hours, but we want to process them in batches for efficiency. This is a perfect use case for buffered channels.
type LogEntry struct {
Timestamp time.Time
Level string
Message string
}
func logGenerator(logs chan<- LogEntry) {
// Simulate incoming logs
for i := 0; i < 10 ; i++{
log := LogEntry{
Timestamp: time.Now(),
Level: []string{"INFO", "WARNING", "ERROR"}[rand.Intn(3)],
Message: fmt.Sprintf("Event #%d occurred", i),
}
logs <- log
time.Sleep(100 * time.Millisecond) // Simulate varying log frequencies
}
close(logs)
}
func logProcessor(logs <-chan LogEntry) {
batch := make([]LogEntry, 0, 3) // Process logs in batches of 3
for log := range logs {
batch = append(batch, log)
if len(batch) == 3 {
// Process batch
processLogBatch(batch)
batch = batch[:0] // Clear the batch
}
}
// Process remaining logs
if len(batch) > 0 {
processLogBatch(batch)
}
}
func processLogBatch(batch []LogEntry) {
fmt.Println("Processing batch of logs:")
for _, log := range batch {
fmt.Printf("[%s] %s: %s\n",
log.Timestamp.Format("15:04:05"),
log.Level,
log.Message)
}
fmt.Println("Batch processing complete\n")
}
func main() {
// Buffer size of 5 to handle burst of logs
logs := make(chan LogEntry, 5)
go logGenerator(logs)
logProcessor(logs)
}
When you run this program, you’ll see output similar to this:
Processing batch of logs:
[14:23:45] INFO: Event #0 occurred
[14:23:45] WARNING: Event #1 occurred
[14:23:45] ERROR: Event #2 occurred
Batch processing complete
Processing batch of logs:
[14:23:46] INFO: Event #3 occurred
[14:23:46] WARNING: Event #4 occurred
[14:23:46] INFO: Event #5 occurred
Batch processing complete
Processing batch of logs:
[14:23:47] ERROR: Event #6 occurred
[14:23:47] INFO: Event #7 occurred
[14:23:47] WARNING: Event #8 occurred
Batch processing complete
Processing remaining logs:
[14:23:48] INFO: Event #9 occurred
Batch processing complete
Understanding the Benefits
This implementation showcases several advanced concepts:
-
Burst Handling: The buffered channel (size 5) can handle bursts of logs without blocking the producer, even if the consumer is busy processing a batch.
-
Batch Processing: Instead of processing each log immediately, we batch them for more efficient processing. This is common in real-world scenarios where batching can reduce database writes or API calls.
-
Graceful Shutdown: The system handles remaining logs before shutting down, ensuring no data is lost.
-
Flexible Processing: The batch size (3) is independent of the channel buffer size (5), allowing us to optimize both independently.
When to Use Buffered Channels
Buffered channels are particularly useful when:
- Your producers and consumers work at different speeds
- You want to batch process items for efficiency
- You need to handle burst of data without blocking producers
- You want to improve performance by reducing synchronization overhead
However, remember that buffered channels don’t solve all problems. They can hide deadlocks and make it harder to reason about your program’s behavior if used incorrectly.
Tips for Success
- Choose buffer sizes carefully - too small negates the benefits, too large can hide problems
- Monitor channel capacity in production using
len(ch)
andcap(ch)
- Consider using contexts for cancellation and timeouts
- Always handle the remaining items when shutting down
What’s Next?
Now that you understand buffered channels and batch processing, you’re ready to explore more advanced patterns like Fan-Out/Fan-In or the Pipeline pattern. Stay tuned for our next article where we’ll dive into those topics!
Conclusion
The Producer-Consumer pattern becomes even more powerful when combined with buffered channels and batch processing. While staying true to Go’s simplicity, these features allow us to build more efficient and resilient systems.
Remember: The key to mastering these patterns is practice. Try modifying the log processing example to handle different batch sizes or add error handling. The possibilities are endless!
Educational Go Patterns by Corentin Giaufer Saubert
is licensed under Creative Commons Attribution-NonCommercial-NoDerivatives 4.0 International
The code examples are licensed under the MIT License.
The banner image has been created by (DALL·E) and is licensed under the same license as the article and other graphics.