Advanced Generator Pattern: Consuming and Testing Data Streams
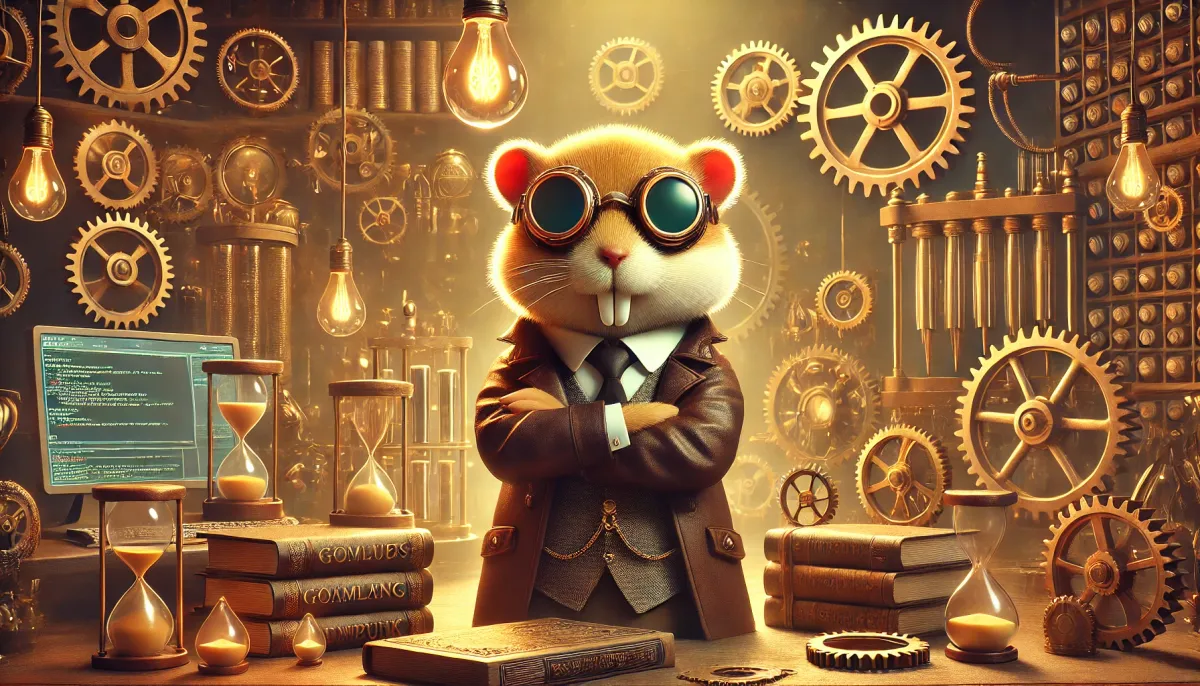
Generator Pattern: Consuming and Testing Data Streams
Difficulty Level: AdvancedIntroduction
Expanding on our previous discussions of the Generator pattern, we’ll explore two advanced applications: consuming large datasets lazily and simulating data streams for testing. These techniques are crucial for efficient data processing and robust application testing.
When to Use
- Processing large datasets that don’t fit in memory
- Simulating data sources for testing
- Implementing ETL (Extract, Transform, Load) processes
- Creating reproducible test scenarios for data processing pipelines
Why to Use
- Memory Efficiency: Process large datasets without loading everything into memory
- Testability: Create controlled environments for testing data processing logic
- Flexibility: Easily switch between real and simulated data sources
- Reproducibility: Generate consistent test cases for data processing scenarios
How it Works
- Create generator functions that yield data items one at a time
- Use channels to stream data from the source to the consumer
- Implement lazy loading for large datasets
- Create mock data generators for testing scenarios
Example 1: Lazy Loading of Large Datasets
type DataItem struct {
ID int
Data string
}
// lazyDataLoader simulates loading a large dataset lazily
func lazyDataLoader(filePath string) <-chan DataItem {
out := make(chan DataItem)
go func() {
defer close(out)
// Simulate opening a large file
fmt.Printf("Opening file: %s\n", filePath)
// Simulate reading the file line by line
for i := 0; i < 1000000; i++ {
// Simulate processing delay for each item
time.Sleep(1 * time.Millisecond)
out <- DataItem{
ID: i + 1,
Data: fmt.Sprintf("Data from line %d", i+1),
}
if i%100000 == 0 {
fmt.Printf("Processed %d items\n", i)
}
}
}()
return out
}
func processData(data <-chan DataItem) {
for item := range data {
// Simulate data processing
processedData := fmt.Sprintf("Processed: %s (ID: %d)", item.Data, item.ID)
fmt.Println(processedData)
}
}
func main() {
dataStream := lazyDataLoader("large_dataset.txt")
processData(dataStream)
}
This example demonstrates lazy loading of a large dataset, processing items one at a time without loading the entire dataset into memory.
Example 2: Simulating Data Streams for Testing
type DataItem struct {
ID int
Data string
}
// mockDataStream simulates a data source (e.g., a file, queue, or network stream)
func mockDataStream(count int) <-chan DataItem {
out := make(chan DataItem)
go func() {
defer close(out)
for i := 0; i < count; i++ {
// Simulate reading from a data source
time.Sleep(100 * time.Millisecond)
out <- DataItem{
ID: i + 1,
Data: fmt.Sprintf("Data-%d", i+1),
}
}
}()
return out
}
// dataGenerator consumes the mock stream and yields processed data
func dataGenerator(stream <-chan DataItem) <-chan string {
out := make(chan string)
go func() {
defer close(out)
for item := range stream {
// Process the data item
processedData := fmt.Sprintf("Processed: %s (ID: %d)", item.Data, item.ID)
out <- processedData
}
}()
return out
}
type StreamGenerator struct{}
func (g StreamGenerator) Execute() {
// Create a mock data stream
dataStream := mockDataStream(10)
// Create a generator to process the stream
processedDataGen := dataGenerator(dataStream)
// Consume and print the processed data
for data := range processedDataGen {
fmt.Println(data)
}
}
This example demonstrates a more structured approach to using the Generator pattern for testing data processing pipelines:
- mockDataStream simulates a data source by generating items with controlled timing
- dataGenerator shows how to process a stream of data items and transform them
- The StreamGenerator type provides a clean interface for executing the pipeline and can be replaced with real data sources in production using DI (Dependency Injection)
- Each stage of the pipeline is clearly separated and testable
Best Practices and Pitfalls
Best Practices:
- Use buffered channels for improved performance when processing large streams
- Implement timeout mechanisms for long-running operations
- Use the
context
package for cancellation in long-running generators - Create configurable mock generators for diverse test scenarios
Pitfalls:
- Not handling errors or edge cases in data generation
- Overlooking resource cleanup in generators (e.g., closing file handles)
- Creating overly complex mock generators that don’t reflect real-world scenarios
- Ignoring performance implications in lazy loading implementations
Summary
The Generator pattern proves invaluable for both consuming large datasets efficiently and creating robust test environments for data processing logic. By leveraging Go’s concurrency features, we can create flexible, memory-efficient, and testable data processing pipelines that can handle real-world scenarios and simulated test cases alike.
Disclaimer
While these examples demonstrate the power of the Generator pattern for data processing and testing, real-world implementations may require additional error handling, resource management, and optimizations. Always consider the specific requirements and constraints of your application when applying these patterns.
For more advanced concurrency patterns and best practices in Go, stay tuned for future articles! 🚀
If you want to experiment with the code examples, you can find them on my GitHub repository.
Educational Go Patterns by Corentin Giaufer Saubert
is licensed under Creative Commons Attribution-NonCommercial-NoDerivatives 4.0 International
The code examples are licensed under the MIT License.
The banner image has been created by (DALL·E) and is licensed under the same license as the article and other graphics.