Flexible Approaches to Worker Pools in Go
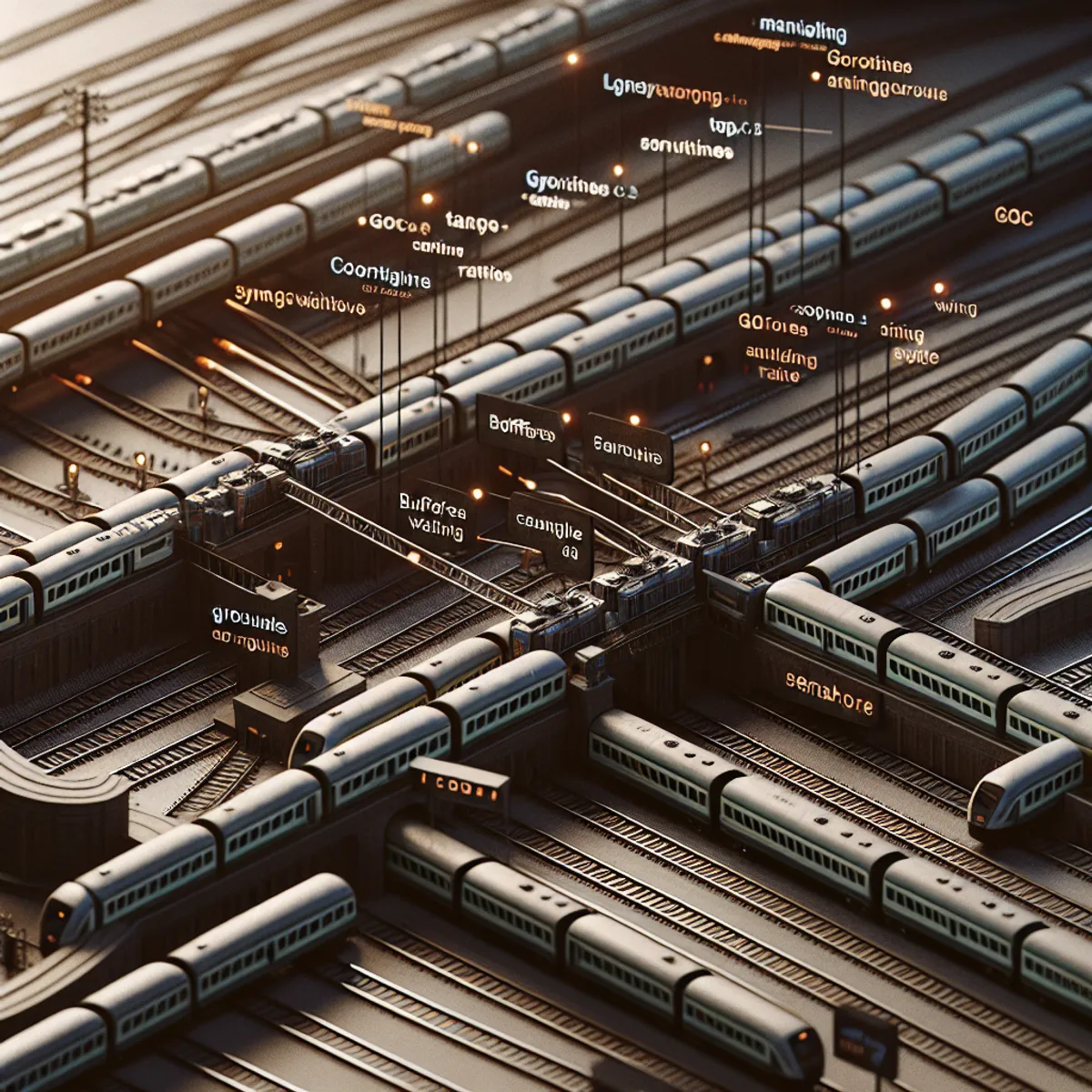
Flexible Approaches to Worker Pools in Go
Difficulty Level: IntermediateIntroduction
While the standard Worker Pool pattern is powerful, Go’s flexibility allows for alternative approaches to concurrent task processing. This article explores the Shared Semaphore method and discusses the use of third-party libraries for managing concurrency in Go applications.
Shared Semaphore Method
The Shared Semaphore method uses a buffered channel as a semaphore to limit concurrency across various parts of an application.
package main
import (
"fmt"
"sync"
"time"
)
func processWithSemaphore(tasks []int, maxConcurrency int) {
sem := make(chan struct{}, maxConcurrency)
var wg sync.WaitGroup
for _, task := range tasks {
wg.Add(1)
sem <- struct{}{} // Acquire semaphore
go func(task int) {
defer wg.Done()
defer func() { <-sem }() // Release semaphore
processTask(task)
}(task)
}
wg.Wait()
}
func processTask(task int) {
fmt.Printf("Processing task %d\n", task)
time.Sleep(time.Second) // Simulate work
fmt.Printf("Completed task %d\n", task)
}
func main() {
tasks := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
processWithSemaphore(tasks, 3)
}
Advantages:
- Simple and lightweight implementation
- Scales to zero when not in use
- Can be used in multiple places without increasing complexity
Use Case:
Ideal for scenarios where you need to limit concurrency across various parts of your application without maintaining a persistent worker pool.
Third-Party Libraries
While implementing your own worker pool is often the best approach, there are some third-party libraries that can be useful in certain situations:
-
Ants: A high-performance goroutine pool in Go, providing features like automatic scaling and reuse of goroutines.
-
sourcegraph/conc: A package for structured concurrency in Go, offering higher-level abstractions for common concurrency patterns.
These libraries can be beneficial when you need advanced features or when working on complex concurrent systems. However, it’s important to note that in approximately 90% of cases, maintaining your own worker pool implementation is preferable. This approach gives you more control, better understanding of your concurrency model, and avoids unnecessary dependencies.
Choosing the Right Approach
Consider the following factors when deciding on your concurrency approach:
- Assess your concurrency needs:
- Do you need to limit concurrency across multiple parts of your application?
- Is your workload consistent or variable?
- Evaluate your task characteristics:
- Are tasks short-lived or long-running?
- Do you need fine-grained control over task execution?
- Consider your application architecture:
- Is simplicity a priority?
- Do you need to scale workers dynamically?
- Decision tree:
- If (need to limit concurrency in specific sections) AND (variable workload): Use Shared Semaphore
- Else if (consistent workload) AND (need fine-grained control): Use Standard Worker Pool
- Else if (need advanced features) AND (complexity is justified): Consider third-party libraries like Ants or sourcegraph/conc
- Else: Implement and maintain your own worker pool
This decision process helps guide your choice based on your specific context and requirements, ensuring you choose the most appropriate concurrency pattern for your needs while prioritizing simplicity and control in most cases.
Summary
While the standard Worker Pool pattern is versatile, Go’s concurrency model allows for flexible approaches like the Shared Semaphore method. This alternative can be particularly useful for applications with varying concurrency needs across different components. Third-party libraries offer advanced features but should be used judiciously, as maintaining your own worker pool often provides the best balance of control and simplicity.
Disclaimer
This article provides an overview of flexible approaches to worker pools in Go. While these patterns are powerful, it’s important to consider the specific needs of your application when implementing them. For production use, additional error handling and optimizations may be necessary.
For more advanced concurrency patterns and best practices in Go, stay tuned for future articles! 🚀
If you want to experiment with the code examples, you can find them on my GitHub repository.
Educational Go Patterns by Corentin Giaufer Saubert
is licensed under Creative Commons Attribution-NonCommercial-NoDerivatives 4.0 International
The code examples are licensed under the MIT License.
The banner image has been created by (DALL·E) and is licensed under the same license as the article and other graphics.